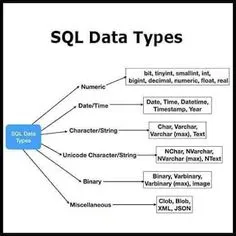
In today’s data-driven world, understanding SQL (Structured Query Language) has become essential for web developers. Databases play a pivotal role in storing, retrieving, and managing data, and SQL is the language used to communicate with these databases. Whether you are a backend or full-stack developer, mastering SQL basics will enhance your ability to work efficiently with data. In this comprehensive guide, we’ll explore the top tips for understanding SQL basics in web development, providing practical examples and best practices along the way.
What Is SQL and Why Is It Important in Web Development?
Before diving into the tips, let’s first define SQL and understand its importance in web development. SQL is a standardized language used to interact with relational databases such as MySQL, PostgreSQL, SQL Server, and Oracle. Its primary function is to retrieve, insert, update, and delete data stored in a database.
For web developers, SQL is critical for backend development because it allows the website to communicate with databases that store essential information, such as user profiles, content, or product catalogs. Without SQL, dynamically generating content, handling user input, and managing data-driven applications would be challenging.
How SQL Powers Web Development
When a user submits a form, clicks a button, or performs a search on a website, an SQL query often runs behind the scenes to fetch the relevant data from the database. SQL also enables developers to optimize website performance by managing large data sets efficiently, ensuring seamless data storage, and handling complex queries.
Let’s explore the top tips for understanding SQL basics in web development, making your journey smoother and more productive.
Understand the Relational Database Model: A Key Concept in SQL
Understanding the relational database model is fundamental to working with SQL in web development. Relational databases organize data into tables, consisting of rows and columns, where each table represents a different entity (like users, products, or orders). These tables are connected through relationships, allowing you to efficiently store, retrieve, and manage related data.
Key components of a relational database include:
- Tables: Data is stored in rows and columns.
- Primary Keys: Unique identifiers for each record.
- Foreign Keys: Links between related tables.
- Indexes: Structures that optimize query performance.
For example, consider the following SQL code that defines a relational database with two related tables, users
and orders
:
CREATE TABLE users (
user_id INT PRIMARY KEY,
name VARCHAR(100),
email VARCHAR(100)
);
CREATE TABLE orders (
order_id INT PRIMARY KEY,
order_date DATE,
user_id INT,
FOREIGN KEY (user_id) REFERENCES users(user_id)
);
In this code, the users
table has a unique user_id
, and the orders
table uses a foreign key to reference the user_id
, creating a relationship between the two tables. This allows you to efficiently manage user and order data, a crucial aspect of modern web applications.
Learn the Core SQL Commands: Essential for Web Development
Mastering the core SQL commands is crucial for working with databases in web development. These basic commands allow you to retrieve, manipulate, and manage data stored in a database, making them indispensable for creating dynamic, data-driven websites. Understanding how to use these commands efficiently will greatly enhance your ability to interact with databases and optimize your backend operations.
Here are the fundamental SQL commands every web developer should know:
- SELECT: Retrieves data from one or more tables.
- INSERT INTO: Adds new records to a table.
- UPDATE: Modifies existing records.
- DELETE FROM: Removes records from a table.
- CREATE TABLE: Defines a new table in the database.
Here’s an example of a simple SQL query using these core commands:
-- Select data from users table
SELECT name, email FROM users;
-- Insert new record into users table
INSERT INTO users (name, email) VALUES ('John Doe', 'john@example.com');
-- Update record in users table
UPDATE users SET email = 'john.doe@example.com' WHERE name = 'John Doe';
-- Delete record from users table
DELETE FROM users WHERE name = 'John Doe';
Optimize SQL Queries for Performance: Boost Your Web Application Speed
Optimizing SQL queries is essential for ensuring your web application runs efficiently, especially when handling large datasets. Poorly written queries can lead to slow page loads and a poor user experience. By optimizing your SQL queries, you can significantly improve database performance and reduce server load, which is critical for scalable web development.
Here are some tips to optimize SQL queries for performance:
- Use SELECT Columns Instead of
SELECT *
: Retrieve only the necessary columns to reduce data transfer. - Create Indexes: Indexes improve query speed by allowing faster data retrieval.
- Limit Results: Use
LIMIT
andOFFSET
to manage large result sets. - Avoid N+1 Queries: Use
JOIN
to retrieve related data in one query.
Example of an optimized SQL query:
-- Optimized query with specific columns and a limit
SELECT product_name, price
FROM products
WHERE category_id = 2
LIMIT 10;
-- Adding an index to improve performance
CREATE INDEX idx_category ON products (category_id);
In this example, selecting only the required columns and limiting the number of results enhances query speed. Adding an index on category_id
further boosts performance, ensuring faster data retrieval for frequently queried fields. By implementing these strategies, your SQL queries will run more efficiently, improving overall application performance.In this example, selecting only the required columns and limiting the number of results enhances query speed. Adding an index on category_id
further boosts performance, ensuring faster data retrieval for frequently queried fields. By implementing these strategies, your SQL queries will run more efficiently, improving overall application performance.
Use Joins to Combine Data from Multiple Tables: A Powerful SQL Technique
In web development, it’s common to store related data across multiple tables. SQL JOIN statements allow you to efficiently combine this data into a single result, making it easier to work with complex datasets. Using joins ensures you can retrieve related information from different tables, like combining user data with their corresponding orders, all in one query.
Here are the key types of SQL Joins:
- INNER JOIN: Returns records that have matching values in both tables.
- LEFT JOIN: Returns all records from the left table, and matched records from the right table.
- RIGHT JOIN: Returns all records from the right table, and matched records from the left table.
- FULL OUTER JOIN: Returns records when there is a match in either table.
Here’s an example of an INNER JOIN
that combines data from two tables:
SELECT users.name, orders.order_date
FROM users
INNER JOIN orders
ON users.user_id = orders.user_id;
In this query, the INNER JOIN
retrieves the name
of users along with their respective order_date
from the orders
table by matching the user_id
. Using joins in your SQL queries is a powerful way to access and display related data efficiently, a key skill for web developers handling multiple database tables.
Handle NULL Values with Care: Ensure Data Integrity in SQL
In SQL, NULL
represents missing or unknown data, and handling it carefully is crucial to avoid unexpected results or errors in your queries. When working with databases in web development, you’ll frequently encounter NULL
values, so it’s important to understand how to manage them to ensure your data remains reliable and consistent.
Here are some key tips for handling NULL
values in SQL:
- Use
IS NULL
orIS NOT NULL
: To filter records with or withoutNULL
values. - Be Cautious with Comparisons: Standard comparison operators (
=
,!=
) don’t work as expected withNULL
. UseIS
for comparisons. - Use Functions Like
COALESCE()
: ReplaceNULL
values with a default value to maintain data consistency.
Example of handling NULL
values:
-- Find records where phone number is missing (NULL)
SELECT name FROM users WHERE phone IS NULL;
-- Replace NULL values with a default value
SELECT name, COALESCE(phone, 'N/A') AS phone_number FROM users;
In the first query, IS NULL
identifies users missing a phone number. The second query uses COALESCE()
to replace NULL
phone numbers with ‘N/A’. Handling NULL
values carefully ensures accurate data representation and avoids issues in your web applications.
Implement Data Validation and Constraints: Ensure Data Accuracy in SQL
Data validation and constraints are crucial for maintaining the accuracy and integrity of your database in web development. SQL provides several built-in constraints that help enforce rules on the data, ensuring only valid information is entered into your tables. By implementing these constraints, you prevent invalid data from entering your database, which can lead to errors and inconsistencies in your web application.
Here are the key types of SQL constraints:
- NOT NULL: Ensures a column cannot store
NULL
values. - UNIQUE: Ensures all values in a column are unique.
- PRIMARY KEY: A combination of
NOT NULL
andUNIQUE
, identifying each record in a table. - FOREIGN KEY: Ensures referential integrity between tables.
- CHECK: Enforces a condition on the data before it’s inserted.
Example of data validation with constraints:
CREATE TABLE users (
user_id INT PRIMARY KEY,
email VARCHAR(255) UNIQUE NOT NULL,
age INT CHECK (age >= 18)
);
In this example, the email
column cannot be NULL
and must be unique, while the age
column enforces a condition that only allows users aged 18 or older. These constraints help ensure the reliability of your data, making your web application more robust and secure.
Learn to Use Subqueries: Simplify Complex SQL Queries
Subqueries, also known as inner queries or nested queries, are an advanced SQL technique that allows you to embed one query inside another. This is especially useful when you need to retrieve or calculate data that depends on the result of another query. Subqueries can greatly simplify complex operations by breaking them into smaller, manageable parts, making your SQL code more readable and efficient.
Here are the key types of subqueries:
- Single-row Subquery: Returns a single value that can be used in conditions.
- Multiple-row Subquery: Returns multiple values, typically used with
IN
orANY
. - Correlated Subquery: A subquery that references columns from the outer query.
Example of a subquery in SQL:
-- Retrieve the names of users who have placed orders with a total greater than 100
SELECT name
FROM users
WHERE user_id IN (SELECT user_id FROM orders WHERE total > 100);
In this example, the subquery retrieves all user_id
s from the orders
table where the total
exceeds 100, and the outer query uses this result to fetch the corresponding name
from the users
table. Subqueries are a powerful tool for performing complex data retrieval tasks in web development, helping you manage databases more effectively.
Use SQL Functions for Data Transformation: Enhance Data Handling in SQL
SQL functions play a vital role in transforming and manipulating data within your database. These built-in functions allow you to perform operations like string manipulation, date calculations, mathematical operations, and aggregations, helping to streamline data processing and improve efficiency in web development. By using SQL functions, you can modify data directly in your queries, reducing the need for additional application logic.
Here are some common SQL functions for data transformation:
- String Functions:
CONCAT()
,UPPER()
,LOWER()
,SUBSTRING()
- Date Functions:
NOW()
,DATE_ADD()
,DATEDIFF()
- Aggregate Functions:
COUNT()
,SUM()
,AVG()
,MAX()
,MIN()
- Math Functions:
ROUND()
,FLOOR()
,CEIL()
Example of SQL functions for data transformation:
-- Convert names to uppercase and round salaries to 2 decimal places
SELECT UPPER(name), ROUND(salary, 2)
FROM employees;
-- Calculate the age of orders in days
SELECT order_id, DATEDIFF(NOW(), order_date) AS order_age
FROM orders;
In these examples, the UPPER()
function transforms names to uppercase, and ROUND()
formats salaries to two decimal places. The DATEDIFF()
function calculates the age of orders in days. Using SQL functions for data transformation enables you to handle and manipulate data more effectively within your web application.
Secure Your SQL Queries Against SQL Injection: Protect Your Database
Securing SQL queries against SQL injection is essential to safeguarding your web application from malicious attacks. SQL injection occurs when attackers manipulate input fields to insert harmful SQL code, potentially gaining unauthorized access to your database. To prevent this, it’s crucial to implement security best practices when writing SQL queries to protect sensitive data and maintain the integrity of your system.
Here are key methods to secure SQL queries:
- Use Prepared Statements: Ensures input values are properly escaped.
- Parameterized Queries: Separates SQL code from user input, preventing malicious SQL injection.
- Input Validation: Always sanitize and validate user inputs before using them in queries.
Example of a secure SQL query using prepared statements:
-- Secure query using prepared statements in PHP
$stmt = $pdo->prepare("SELECT * FROM users WHERE email = ?");
$stmt->execute([$email]);
In this example, the prepared statement ensures that the input for email
is treated as data rather than executable code. By using prepared statements, parameterized queries, and validating inputs, you can effectively secure your SQL queries against SQL injection attacks, protecting your web application and database from potential breaches.
Practice Writing and Testing SQL Queries: Boost Your SQL Skills
Consistent practice in writing and testing SQL queries is key to mastering SQL and improving your web development capabilities. Writing efficient queries and testing them in real scenarios helps you understand database structures, optimize performance, and handle data effectively. Regularly practicing SQL enables you to refine your skills, avoid common mistakes, and develop a deeper understanding of how to interact with databases.
Here are ways to enhance your SQL practice:
- Use SQL Sandboxes: Tools like SQLFiddle or db<>fiddle allow you to experiment with queries.
- Test Queries with Sample Data: Set up local databases and test queries to ensure accuracy.
- Debug and Optimize: Analyze query performance and debug issues with SQL testing environments like MySQL Workbench.
Example of a query to practice:
-- Retrieve top 5 customers based on total orders
SELECT name, COUNT(order_id) AS total_orders
FROM customers
JOIN orders ON customers.customer_id = orders.customer_id
GROUP BY name
ORDER BY total_orders DESC
LIMIT 5;
In this example, the query retrieves the top 5 customers based on the number of orders placed. Regularly practicing and testing queries like this helps you identify patterns, troubleshoot issues, and optimize your SQL code for better performance in real-world web development scenarios.
Conclusion
Mastering the top tips for understanding SQL basics in web development is essential for building robust, data-driven websites and applications. From understanding the relational database model to optimizing queries and securing your code against SQL injection, these tips provide a strong foundation for working effectively with databases. By applying these strategies and continuing to practice, you’ll gain the confidence and skills needed to excel in your web development career.